I usually use Rspec with Capybara, in order to create feature tests. These test views with a real browser. And sometimes we have triggering timeouts, and random failures in tests, due to slow javascripts executions, random load in test cases ….
11 years ago now, Jonas Nicklas, Capybara’s leader, removed wait_until from the code. Basically it introduced an abuse of this clause in our tests, and random execution times. Here you can read some well explained reasons, with the basic recommendatio to reasearch why are happenning these timeouts instead of waiting long times in your test suites. Delay can be huge when you have a good amount of tests.
But Capybara introduced some wait clauses tha I will explain below….
You can increate wait time in capybara assertions at `spec/rails_helper.rb`:
Capybara.default_max_wait_time = 10 # default is 2 |
Capybara.default_max_wait_time = 10 # default is 2
Sometimes developers use `sleep 0.5` on their code. Problem here is that they are fixed waits, opposite than ‘wait’ or ‘wait_until’ which exit when a condition is achieved.
As alternative wyou can use `wait:` options in all contions like `have_….`:
expect(page).to have_text(I18n.t('investments.sale_movements.form.title'), wait: 20) |
expect(page).to have_text(I18n.t('investments.sale_movements.form.title'), wait: 20)
This option waits the amount of 20 second, then, fails:
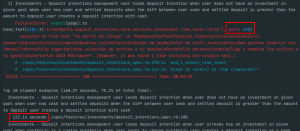
In case you are not using a Capybara assertion, you can use the following block:
using_wait_time 60 do
expect(Organization.find_by(subdomain: organization.subdomain)).to be_present
end |
using_wait_time 60 do
expect(Organization.find_by(subdomain: organization.subdomain)).to be_present
end
These two last cases modify the default time inculded in configuration file.
This does not remove all failures, but it improves legibility. And you can check in tests logs if test is failing by a timeout or antother reason.
Happy coding!!!